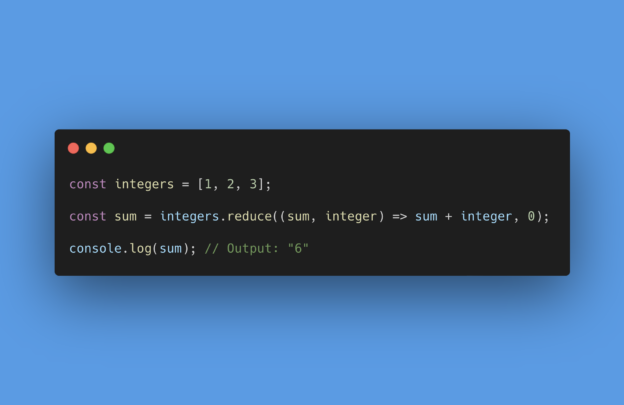
Reduce in TypeScript
The basic idea of the Array.prototype.reduce() function is to reduce an array to a single value. For example, reduce an array of integers into their combined sum.
In other words, instead of calculating the sum of an array of numbers like:
const integers = [1, 2, 3]; let sum = 0; for (let i = 0; i < integers.length; i++) { sum += integers[i]; } console.log(sum); // Output: "6"
Then, reduce can be used to sum an array of integers like this:
const integers = [1, 2, 3]; const sum = integers.reduce((sum, integer) => sum + integer, 0); console.log(sum); // Output: "6"
When using reduce, there are three key elements involved:
- An array with elements that should be reduced to a value, such as the integers array in the example above.
- An initial value of the variable that will eventually be returned as the result of the reduce function. The initial value is 0 in the sum example above.
- A function that is called for each element in the array, so in the sum example above it would be called three times. The function has two parameters:
- previousValue (renamed to sum in the example above): The accumulated result of the processing so far. When the function is called on the first element in the array, then previousValue has the value specified in initial value (0 in the example above). After that previousValue will hold the return value of the previous element that the function was called for.
- currentValue (renamed to integer in the example above): The array element that is currently being processed. The function will be called once for each element in the array.
- Return value: The function should return the new accumulated result of the processing. The return value will then be provided in the previousValue parameter when processing the next element in the array.
As can be seen in the documentation there are more optional parameters, but one can get pretty far with the parameters above.
π‘ Tip 1: Leave out the initial value
When calling reduce, the initial value can be left out and then the first array element is used as the initial value. For example, to join an array of strings:
const titles = ['Clean TypeScript', 'Stateless React', 'Functional Core']; const joinedTitles = titles.reduce((joinedTitles, title) => joinedTitles += `, ${title}`); console.log(joinedTitles); // Output: "Clean TypeScript, Stateless React, Functional Core"
I tend not to use this, because if it is called on an empty array, then it will fail. TypeScript can of course catch those instances on compile time where this might happen but cannot catch those that originate from an external API or database.
π‘ Tip 2: Rename the parameters to match your function
The function given as input to reduce is using previousValue and currentValue as default names of its parameters. It’s almost always a good idea to rename these parameters to something more precise and fitting for the domain of the function.
π‘ Tip 3: Start with defining the return value
If it is a reduce function with some complex processing, I tend to start with the return value by defining the initial value and then work backwards from that.
π‘ Tip 4: Learning by doing
Reduce can be a bit more difficult to learn than map, filter, and the other array functions, and I think the best way to learn reduce is simply to copy/paste some of the examples from this post (and from my post with reduce examples) into TypeScript Playground to get some hands-on experience, learning-by-doing and all that.
In the next post, I will add a number of examples of using Reduce to solve a number of common scenarios. Stay tuned!π